Styling the ActionBar – Part 3
Previously in this series we have applied a few simple styles to the ActionBar. In this article we’ll have a look at styling the overflow menu and the Navigation DropDown.
Let’s begin with the overflow menu. We must first define some new styles inres/values/styles.xml for the menu panel and the ListView selector:
For this we need to create a new XML drawable inres/drawable/selectable_background_stylingactionbar.xml
This requires list_focused_stylingactionbar.9.png to be copied across from each of the drawable-hdpi, drawable-mdpi, & drawable-xhdpi directories in the zip that we downloaded from Jeff Gilfelt’s ActionBar Style Generator tool. Now we need to create another XML drawable in res/drawable/pressed_background_stylingactionbar.xml:
We also need to create a new colour in res/drawable/colors.xml:
Next we need to copy across menu_dropdown_panel_stylingactionbar.9.png from the drawable-hdpi, drawable-mdpi, & drawable-xhdpi directories in the ActionBar Style Generator zip to their respective directories in our project.
Finally, we need to add the styles that we have created to the theme so they are applied at runtime:
If we run this we would expect the overflow menu to be correctly styled, but in fact it is not:
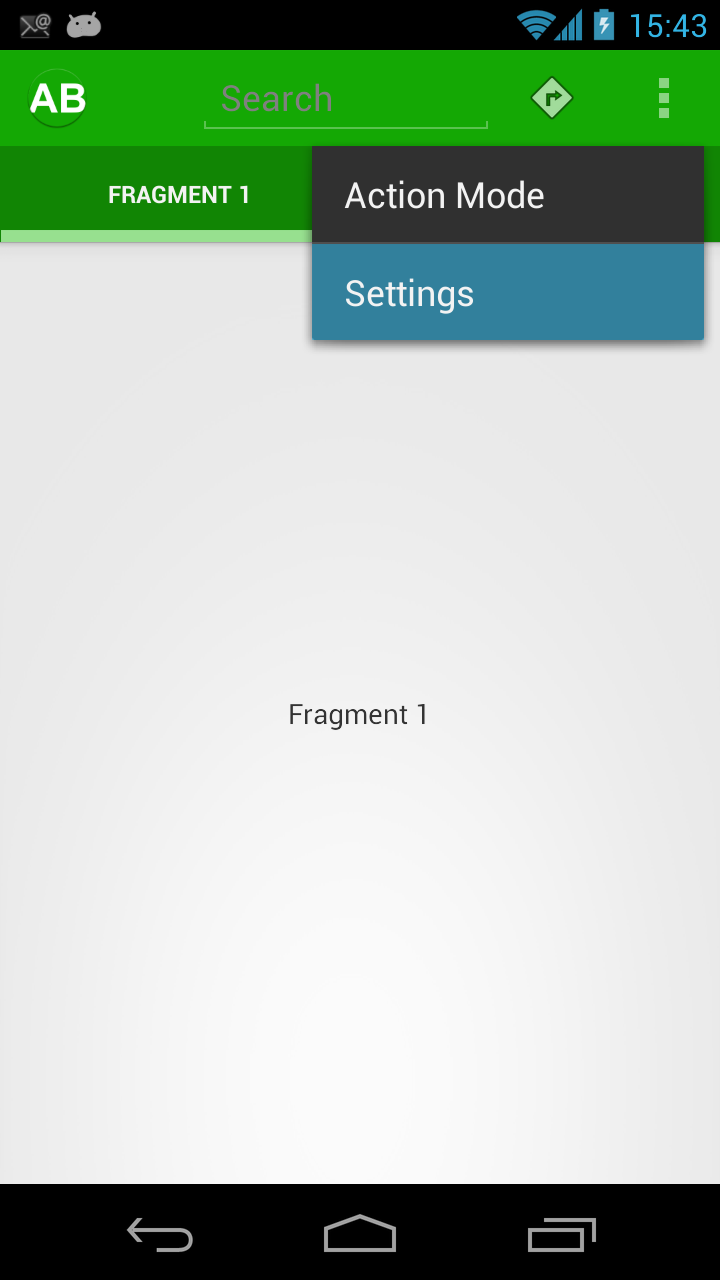
So, what is going wrong here? Actually this would work perfectly if we were using one of the standard themes such as Theme.Holo.Light, but in our case the styling is not happening because we are using Theme.Holo.Light.DarkActionBar as the basis of our theme. This theme uses a bit of trickery to get the darker colour working, and so we need to use a bit of trickery also. It’s actually not that difficult, and utilises the styles that we have already created, we just need to apply them slightly differently in our theme.
One thing that we’ll come on to later is how to apply styles to MenuItems where we are using a actionViewClass or actionViewLayout. In fact each of the MenuItems can have styles applied, and the overflow menu is no different. What we need to do is to create a new theme which applies the styles that we have already configured:
Then apply this to the widget style in our main theme:
Now we can run it again, and the styling has now been correctly applied to the overflow menu:
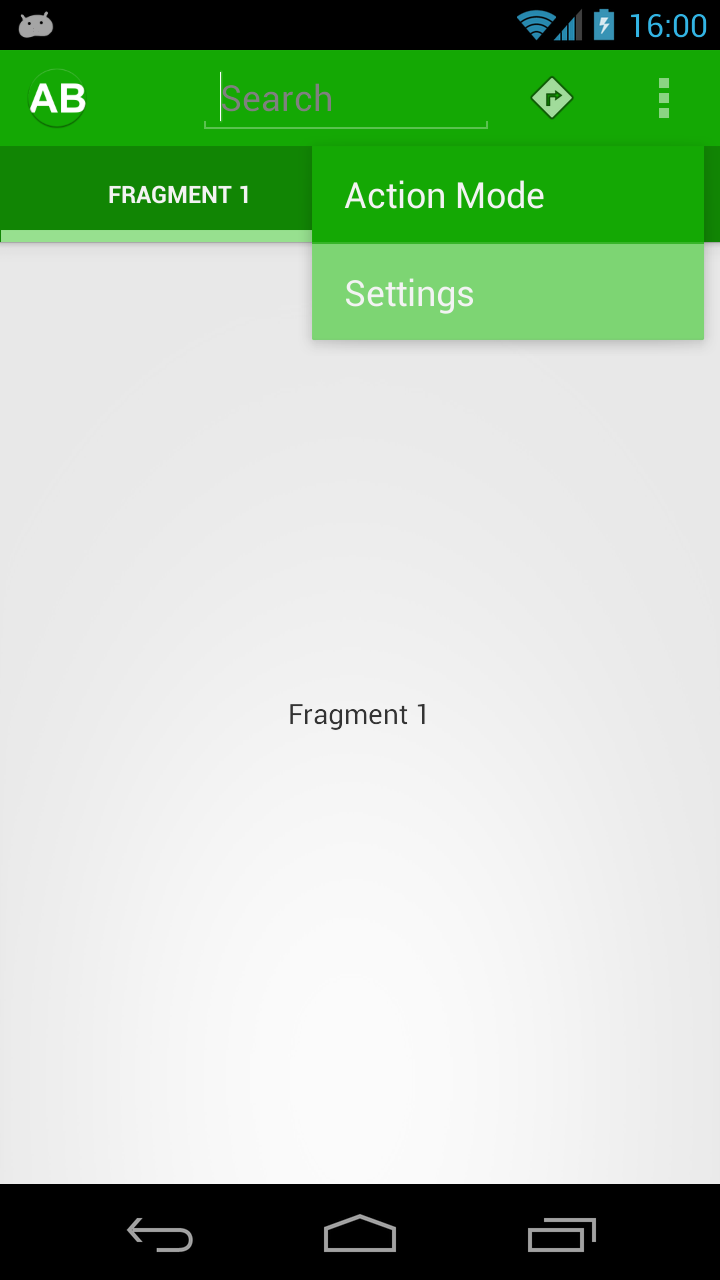
Next we’ll look at the Spinner used for DropDown Navigation. What we need to do is pretty similar to what we’ve done before. We need to create a new style:
Then we copy across spinner_background_ab_stylingactionbar.xml from drawable in the ActionBar Style Generator zip to drawable in our projects, and then copy spinner_ab_default_stylingactionbar.9.png, spinner_ab_disabled_stylingactionbar.9.png, spinner_ab_focused_stylingactionbar.9.png, & spinner_ab_pressed_stylingactionbar.9.png from each of the drawable-hdpi, drawable-mdpi, & drawable-xhdpi folders in the zip to their respective folders in our project. Finally we apply this style in our theme:
Once again we run this:
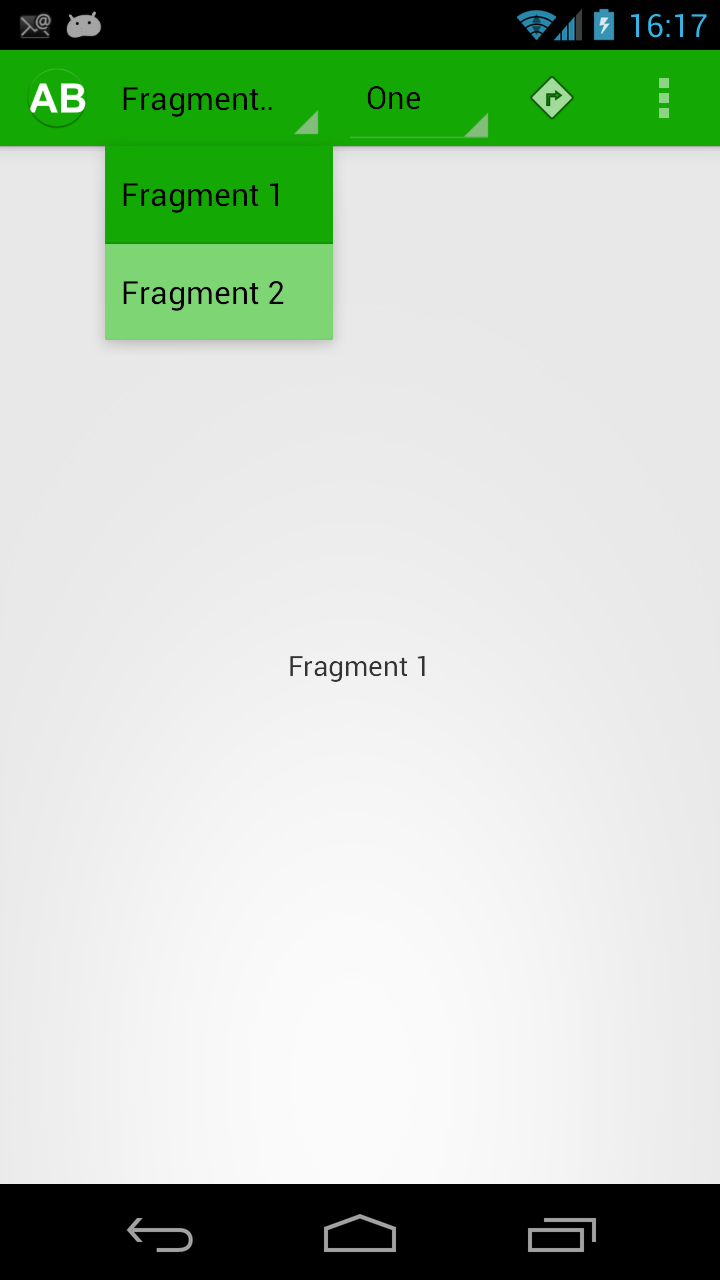
This is looking pretty good, but to stay in keeping with the rest of the ActionBar styling we really want white text here. This is easy enough to achieve by creating a couple of new styles which apply the inverse text colour (because we’re usingTheme.Holo.Light.DarkActionBar as our base) to both the text of the Spinner itself and the text of the DropDown:
Once again we apply these styles in our theme:
If we run this, we can see that we now have the correcty coloured text:
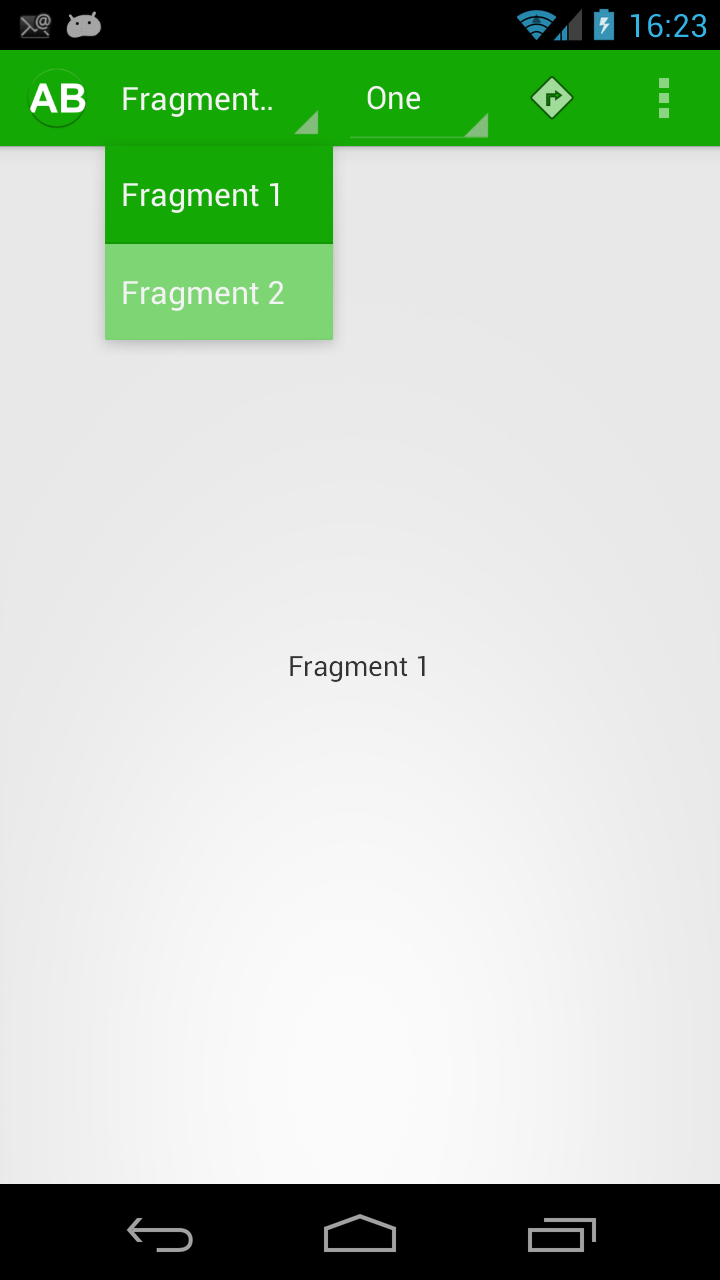
Everything looks good until we look at the second Spinner that we created:
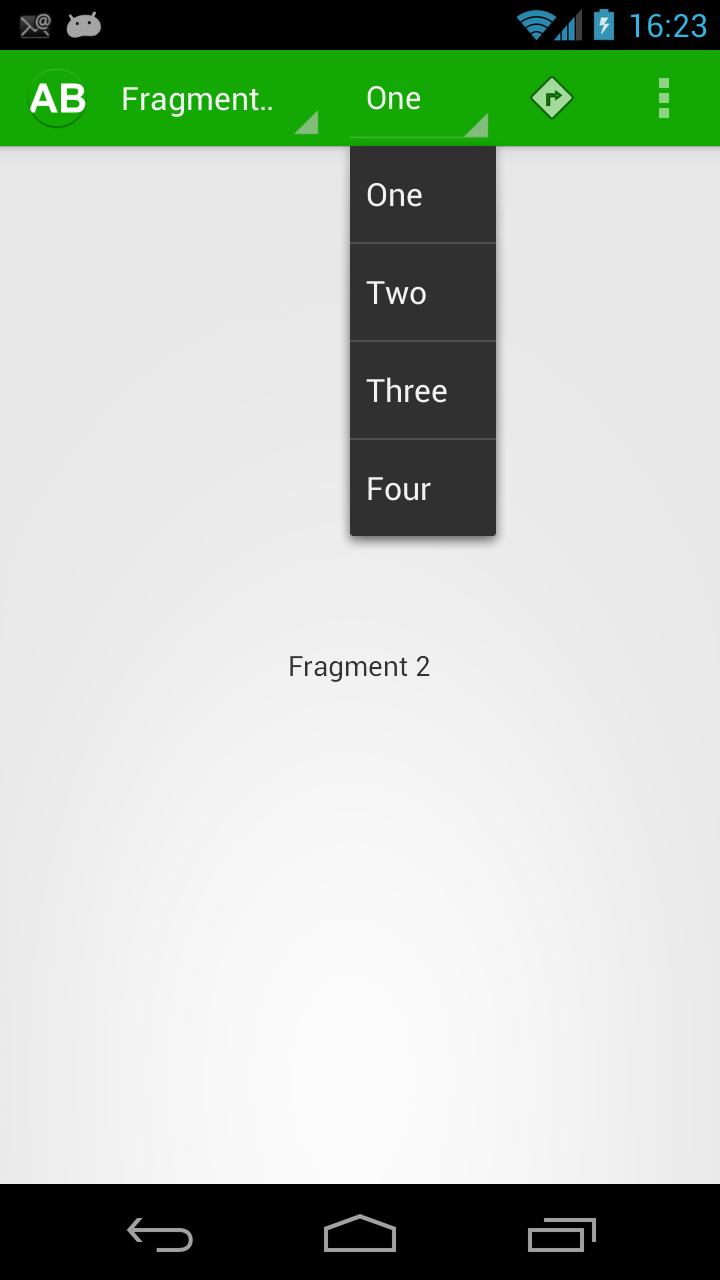
In the next part of this series we’ll examine why our style hasn’t been fully applied to the second Spinner drop down, and get this properly styled.
Let’s begin with the overflow menu. We must first define some new styles inres/values/styles.xml for the menu panel and the ListView selector:
1
2
3
4
5
6
7
| < style name = "PopupMenu" parent = "@android:style/Widget.Holo.ListPopupWindow" > < item name = "android:popupBackground" >@drawable/menu_dropdown_panel_stylingactionbar</ item > </ style > < style name = "DropDownListView" parent = "@android:style/Widget.Holo.ListView.DropDown" > < item name = "android:listSelector" >@drawable/selectable_background_stylingactionbar</ item > </ style > |
For this we need to create a new XML drawable inres/drawable/selectable_background_stylingactionbar.xml
1
2
3
4
5
6
7
8
9
10
| <? xml version = "1.0" encoding = "utf-8" ?> android:exitFadeDuration = "@android:integer/config_mediumAnimTime" > < item android:state_pressed = "false" android:state_focused = "true" android:drawable = "@drawable/list_focused_stylingactionbar" /> < item android:state_pressed = "true" android:drawable = "@drawable/pressed_background_stylingactionbar" /> < item android:drawable = "@android:color/transparent" /> </ selector > |
1
2
3
4
5
| <? xml version = "1.0" encoding = "utf-8" ?> android:shape = "rectangle" > < solid android:color = "@color/pressed" /> </ shape > |
1
| < color name = "pressed" >#CC97e08f</ color > |
Finally, we need to add the styles that we have created to the theme so they are applied at runtime:
1
2
3
4
5
6
| < style name = "AppTheme" parent = "android:Theme.Holo.Light.DarkActionBar" > < item name = "android:actionBarStyle" >@style/ActionBar</ item > < item name = "android:actionBarTabStyle" >@style/ActionBarTabStyle</ item > < item name = "android:popupMenuStyle" >@style/PopupMenu</ item > < item name = "android:dropDownListViewStyle" >@style/DropDownListView</ item > </ style > |
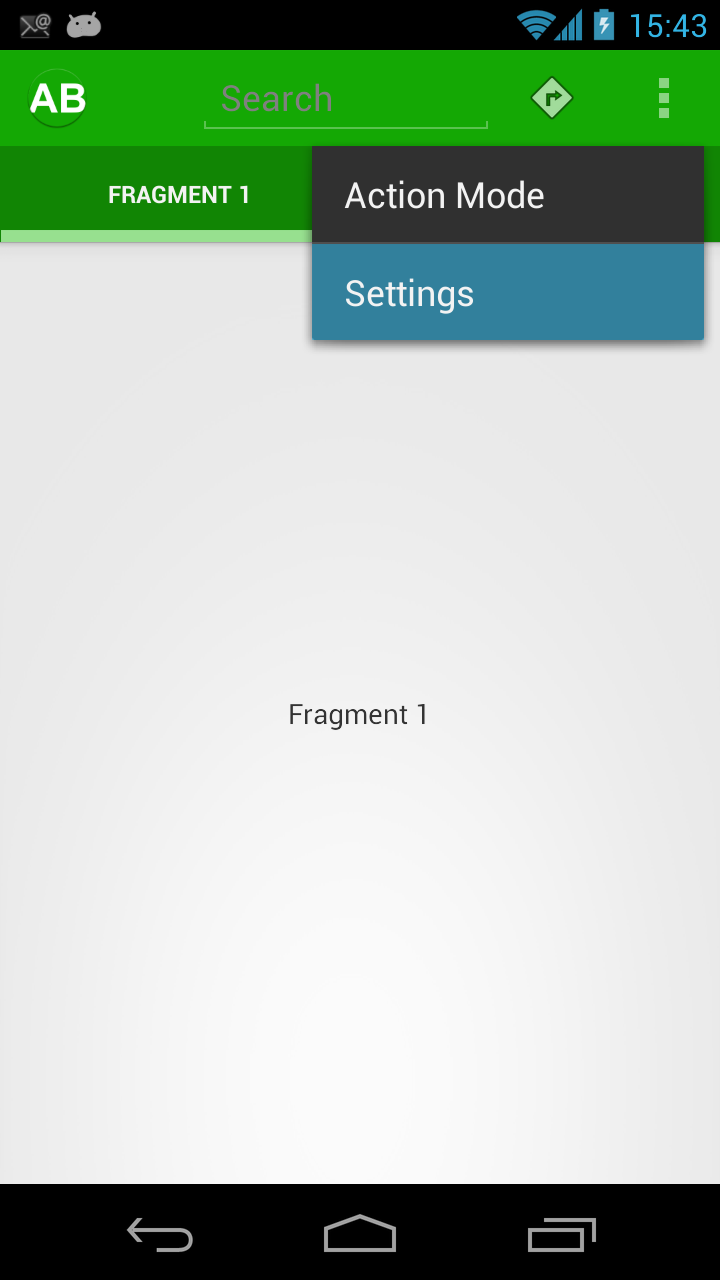
So, what is going wrong here? Actually this would work perfectly if we were using one of the standard themes such as Theme.Holo.Light, but in our case the styling is not happening because we are using Theme.Holo.Light.DarkActionBar as the basis of our theme. This theme uses a bit of trickery to get the darker colour working, and so we need to use a bit of trickery also. It’s actually not that difficult, and utilises the styles that we have already created, we just need to apply them slightly differently in our theme.
One thing that we’ll come on to later is how to apply styles to MenuItems where we are using a actionViewClass or actionViewLayout. In fact each of the MenuItems can have styles applied, and the overflow menu is no different. What we need to do is to create a new theme which applies the styles that we have already configured:
1
2
3
4
| < style name = "Theme.stylingactionbar.widget" parent = "@android:style/Theme.Holo" > < item name = "android:popupMenuStyle" >@style/PopupMenu</ item > < item name = "android:dropDownListViewStyle" >@style/DropDownListView</ item > </ style > |
1
2
3
4
5
6
7
| < style name = "AppTheme" parent = "android:Theme.Holo.Light.DarkActionBar" > < item name = "android:actionBarStyle" >@style/ActionBar</ item > < item name = "android:actionBarTabStyle" >@style/ActionBarTabStyle</ item > < item name = "android:popupMenuStyle" >@style/PopupMenu</ item > < item name = "android:dropDownListViewStyle" >@style/DropDownListView</ item > < item name = "android:actionBarWidgetTheme" >@style/Theme.stylingactionbar.widget</ item > </ style > |
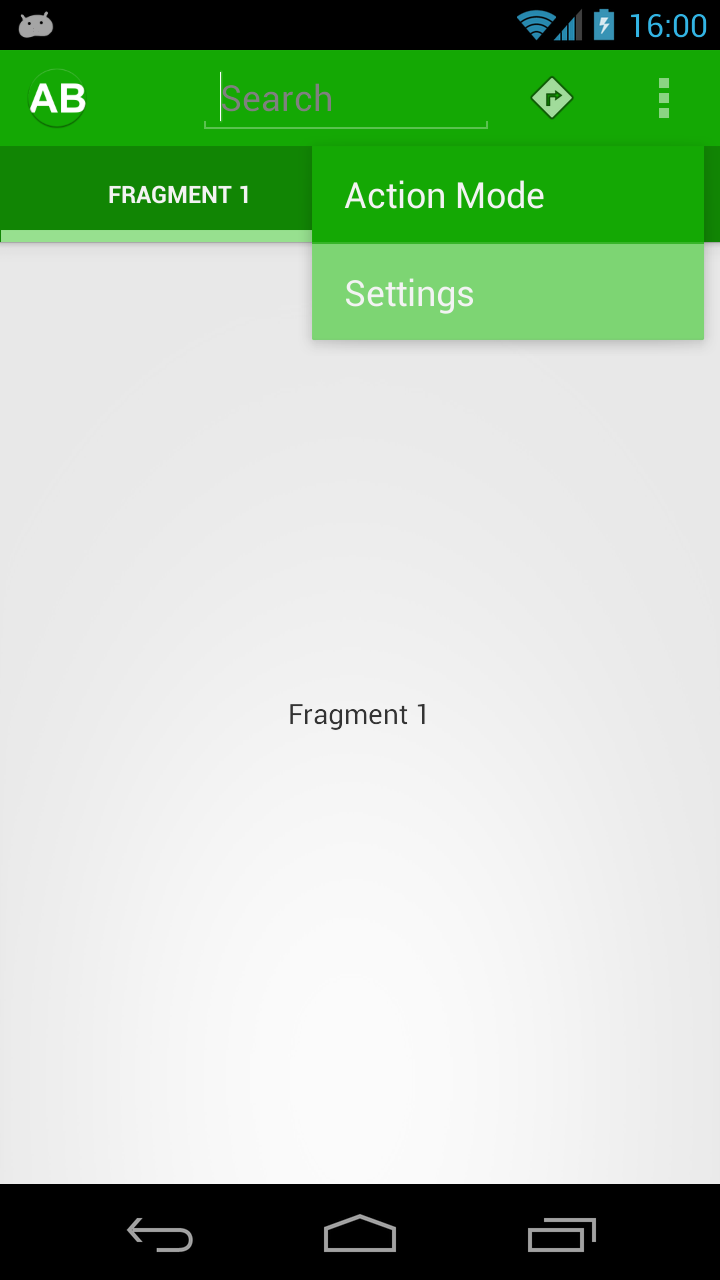
Next we’ll look at the Spinner used for DropDown Navigation. What we need to do is pretty similar to what we’ve done before. We need to create a new style:
1
2
3
4
5
| < style name = "DropDownNav" parent = "@android:style/Widget.Holo.Spinner" > < item name = "android:background" >@drawable/spinner_background_ab_stylingactionbar</ item > < item name = "android:popupBackground" >@drawable/menu_dropdown_panel_stylingactionbar</ item > < item name = "android:dropDownSelector" >@drawable/selectable_background_stylingactionbar</ item > </ style > |
1
2
3
4
5
6
7
8
| < style name = "AppTheme" parent = "android:Theme.Holo.Light.DarkActionBar" > < item name = "android:actionBarStyle" >@style/ActionBar</ item > < item name = "android:actionBarTabStyle" >@style/ActionBarTabStyle</ item > < item name = "android:popupMenuStyle" >@style/PopupMenu</ item > < item name = "android:dropDownListViewStyle" >@style/DropDownListView</ item > < item name = "android:actionBarWidgetTheme" >@style/Theme.stylingactionbar.widget</ item > < item name = "android:actionDropDownStyle" >@style/DropDownNav</ item > </ style > |
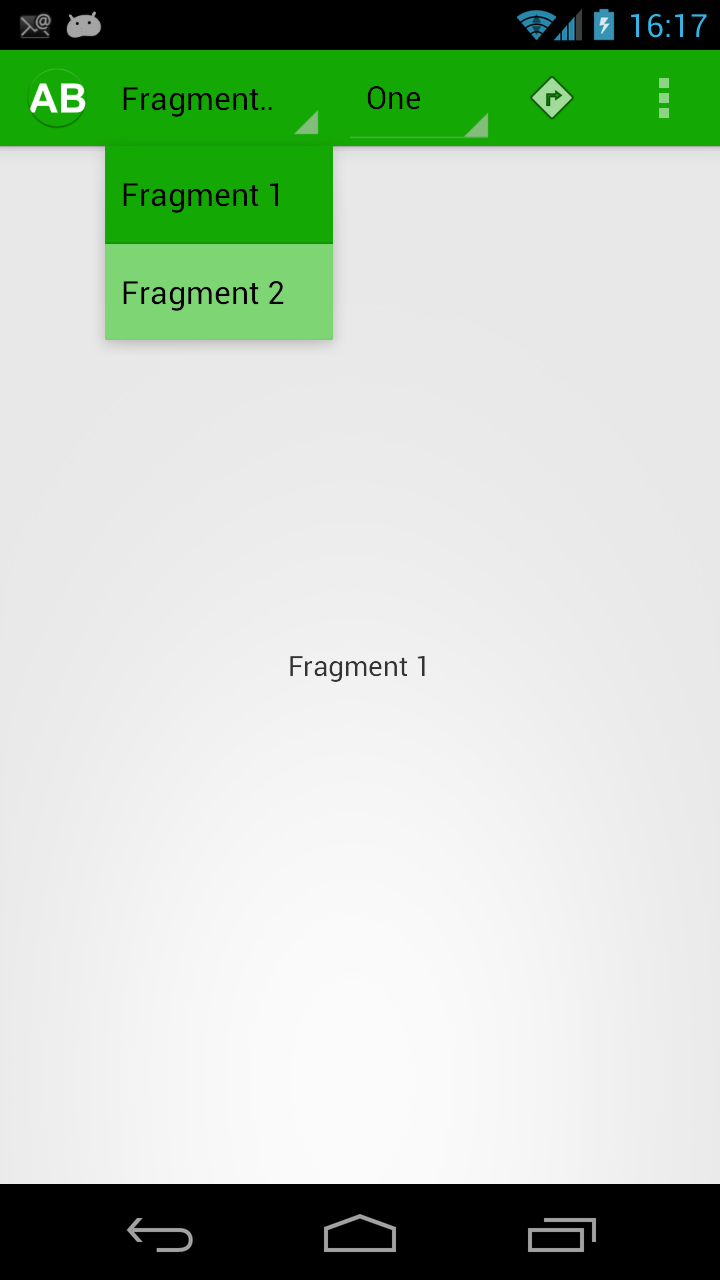
This is looking pretty good, but to stay in keeping with the rest of the ActionBar styling we really want white text here. This is easy enough to achieve by creating a couple of new styles which apply the inverse text colour (because we’re usingTheme.Holo.Light.DarkActionBar as our base) to both the text of the Spinner itself and the text of the DropDown:
1
2
3
4
5
6
7
| < style name = "DropDownItem" parent = "@android:style/Widget.Holo.Light.DropDownItem.Spinner" > < item name = "android:textAppearance" >?android:attr/textAppearanceInverse</ item > </ style > < style name = "SpinnerItem" parent = "@android:style/Widget.Holo.TextView.SpinnerItem" > < item name = "android:textAppearance" >?android:attr/textAppearanceInverse</ item > </ style > |
1
2
3
4
5
6
7
8
9
10
| < style name = "AppTheme" parent = "android:Theme.Holo.Light.DarkActionBar" > < item name = "android:actionBarStyle" >@style/ActionBar</ item > < item name = "android:actionBarTabStyle" >@style/ActionBarTabStyle</ item > < item name = "android:popupMenuStyle" >@style/PopupMenu</ item > < item name = "android:dropDownListViewStyle" >@style/DropDownListView</ item > < item name = "android:actionBarWidgetTheme" >@style/Theme.stylingactionbar.widget</ item > < item name = "android:actionDropDownStyle" >@style/DropDownNav</ item > < item name = "android:spinnerDropDownItemStyle" >@style/DropDownItem</ item > < item name = "android:spinnerItemStyle" >@style/SpinnerItem</ item > </ style > |
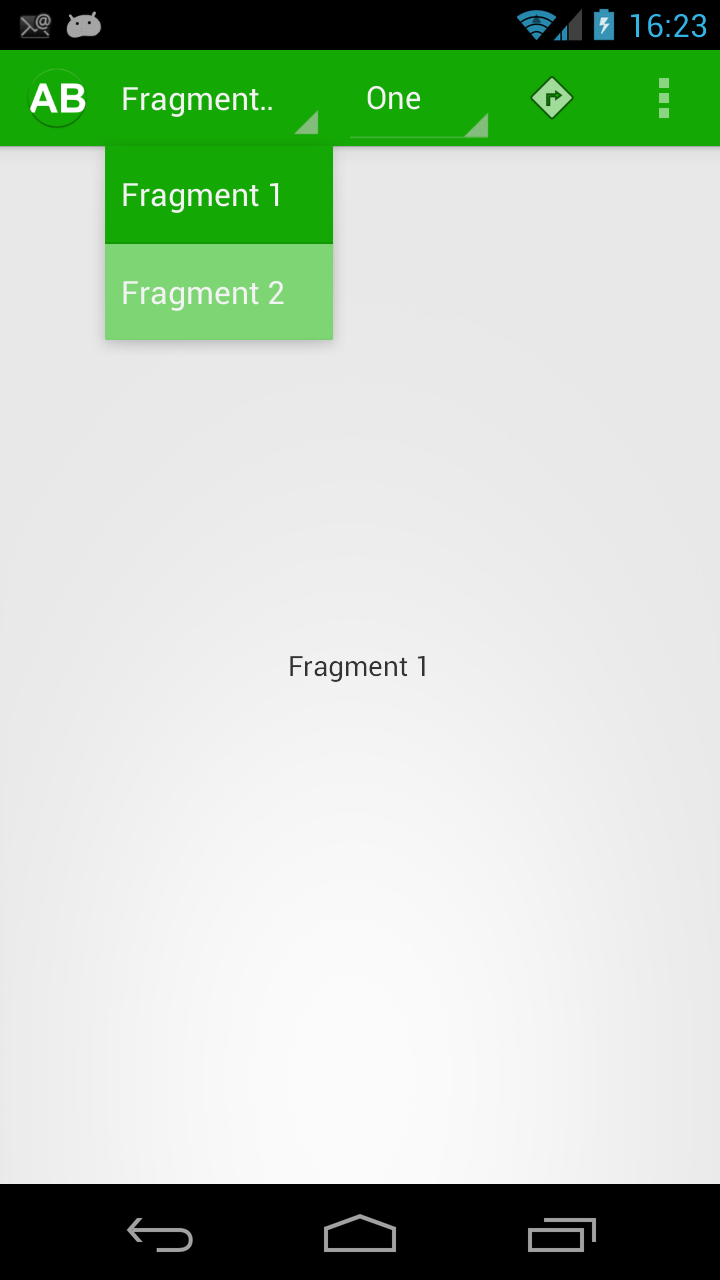
Everything looks good until we look at the second Spinner that we created:
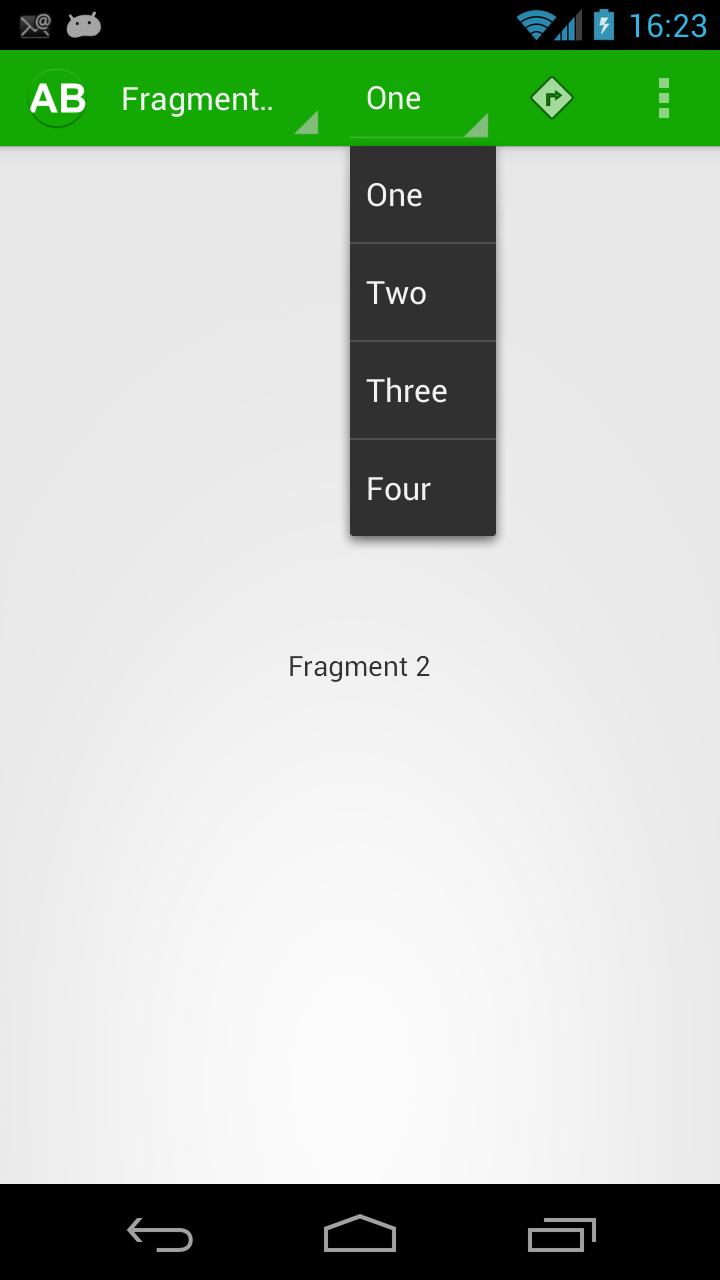
In the next part of this series we’ll examine why our style hasn’t been fully applied to the second Spinner drop down, and get this properly styled.
No hay comentarios:
Publicar un comentario